Your assignment is to implement simple distance vector routing algorithm.
Distance vectors:
Each node (implemented as a java thread) will have a distance vector, which is a list of distance vector entries, one for each node in the network. The distance vector stored at A should contain A's best path for all destinations with the cost and the next hop to take for a destination and the time at which this entry was last updated.
1. The next hop for A to send the packet for the destination Z.
2. The cost of going to Z
3. The time at which this entry was last updated.
When a node reboots, it should initialize its distance vector. Example: Consider the following network,
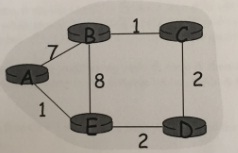
Initialization at node A:
Nodes A B C D E
Cost 0 infinity infinity infinity infinity
Nexthop - - - - -
Update 0 0 0 0 0
After 1st iteration:
Nodes A B C D E
Cost 0 7 infinity infinity 1
Nexthop - B - - E
Update 0 x 0 0 x
After 2nd iteration:
Nodes A B C D E
Cost 0 7 8 infinity 1
Nexthop - B B - E
Update 0 x y 0 x
Updates:
Every once in a while (you are free to decide how often), each node should send an update along each of its links. An update sent by node A should contain A's entire distance vector. An update could contain other information if you want it to.
Before A sends an update, it should check its distance vector for old entries. Any entry that has not been updated for a while (again, you decide how long) should be eliminated. That is. the entry's guess at the number of hops between A and the node in question should be set to infinity.
Upon receiving an update from a neighboring node B, node A should compare each entry in the update with the corresponding entry in A's distance vector. Node A should change its entry for destination C if either
1. (the hops from B to C) + 1 < (the hops from A to C), and the first node on the route from B to C is not A.
OR
2. the first node on the route from A to C is B
Print out DV of each node after an update.